ES6 Modules
Modules let you write componentized and sharable code. Without complete support from any browser, developers have already started to get a taste of ES6 modules through transpiling from tools such as TypeScript or Babel. Edge now has early support for ES6 modules behind an experimental flag.
Modules aren’t exactly new to JavaScript with formats such as Asynchronous Module Definition (AMD) and CommonJS predating ES6. The key value of having modules built in to the language is that JavaScript developers can easily consume or publish modular code under one unified module ecosystem that spans both client and server. ES6 modules feature a straightforward and imperative-style syntax, and also have a static structure that paves the way for performance optimizations and other benefits such as circular dependency support. There are times when modules need to be loaded dynamically, for example some modules might only be useful and thus loaded if certain conditions are met in execution time. For such cases, there will be a module loader which is still under discussion in the standardization committee and will be specified/ratified in the future.
ES6 Modules in Microsoft Edge
To light up ES6 modules and other experimental JavaScript features in Edge, you can navigate to about:flags and select the “Enable experimental JavaScript features” flag.
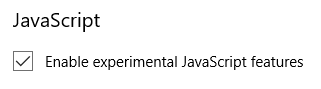
As a first step, Edge and Chakra now support all declarative import/export syntax defined in ES6 with the exception of namespace import/export (import * as name from “module-name” and export * from “module-name”). To load modules in a page, you can use the <script type=”module”> tag. Here is an example of using a math module:
[code language=”html”]
/* index.html */
…
<script type=’module’ src=’./app.js’>
…
[/code]
[code language=”javascript”]
/* app.js */
import { sum } from ‘./math.js’;
console.log(sum(1, 2));
/* math.js */
export function sum(a, b) { return a + b; }
export function mult(a, b) { return a * b; }
[/code]
Implementation: static modules = faster lookup
One of the best aspects of ES6’s modules design is that all import and export declarations are static. The spec syntactically restricts all declarations to the global scope in the module body (no imports/exports in if-statement, nested function, eval, etc.), so all module imports and exports can be determined during parsing and will not change in execution.
From an implementation perspective, Chakra takes advantage of the static nature in several ways, but the key benefit is to optimize looking up values of import and export binding identifiers. In Microsoft Edge, after parsing the modules, Chakra knows exactly how many exports a module declares and what they are all named, Chakra can allocate the physical storage for the exports before execution. For an import, Chakra resolves the import name and create a link back to the export it refers to. The fact that Chakra is aware of the exact physical storage location for imports and exports allows it to store the location in bytecode and skip dynamically looking up the export name in execution. Chakra can bypass much of the normal property lookup and cache mechanisms and instead directly fetch the stored location to access imports or exports. The end result is that working with an imported object is faster than looking up properties in ordinary objects.
Going forward with modules
We have taken an exciting first step to support ES6 modules. As the experimental status suggests, the work towards turning ES6 modules on by default in Edge is not fully done yet. Module debugging in the F12 Developer Tools is currently supported in internal builds and will be available for public preview soon. Our team is also working on namespace import/export to have the declarative syntax fully supported and will look into module loader once its specification stabilizes. We also plan to add new JSRT APIs to support modules for Chakra embedders outside of Edge.
More ES6 Language Features
Microsoft Edge has led the way on a number of ES6 features. Chakra has most of ES6 implemented and on by default including the new syntax like let and const, classes, arrow functions, destructuring, rest and spread parameters, template literals, and generators as well as all the new built-in types including Map, Set, Promise, Symbol, and the various typed arrays.
In current preview builds, there are two areas of ES6 that are not enabled by default – well-known symbols and Proper Tail Calls. Well-known symbols need to be performance optimized before Chakra can enable them – we look forward to delivering this support in an upcoming Insider flight later this year.
The future of Proper Tail Calls, on the other hand, is somewhat in doubt – PTC requires a complex implementation which may result in performance and standards regressions in other areas. We’re continuing to evaluate this specification based on our own implementation work and ongoing discussions at TC39.
ES2016 & Beyond with the New TC39 Process
TC39, the standards body that works on the ECMAScript language, has a new GitHub-driven process and yearly release cadence. This new process has been an amazing improvement so far for a number of reasons, but the biggest is that it makes it easier for implementations to begin work early for pieces of a specification that are stable and specifications are themselves much smaller. As such, Chakra got an early start on ES2016 and are now shipping a complete ES2016 implementation. ES2016 was finalized (though not ratified) recently and includes two new features: the exponentiation operator and Array.prototype.includes.
The exponentiation operator is a nice syntax for doing Math.pow
and it uses the familiar **
syntax as used in a number of other languages. For example, a polynomial can be written like:
[code language=”javascript”]let result = 5 * x ** 2 – 2 * x + 5;[/code]
Certainly a nice improvement over Math.pow, especially when more terms are present.
Chakra also supports Array.prototype.includes which is a nice ergonomic improvement over the existing Array.prototype.indexOf method. Includes returns true
or false
rather than an index which makes usage in Boolean contexts lots easier. includes
also handles NaN properly, finally allowing an easy way to detect if NaN is present in an array.
Meanwhile, the ES2017 specification is already shaping up and Chakra has a good start on some of those features as well, namely Object.values and entries, String.prototype.padStart and padEnd, and SIMD. We’ve blogged about SIMD in the past, though recently Chakra has made progress in making SIMD available outside of asm.js. With the exception of Object.values and entries, these features are only available with experimental JavaScript features flag enabled.
Object.values & Object.entries are very handy partners to Object.keys. Object.keys gets you an array of keys of an object, while Object.values gives you the values and Object.entries gives you the key-value pairs. The following should illustrate the differences nicely:
[code language=”javascript”]let obj = { a: 1, b: 2 };
Object.keys(obj);
// [ ‘a’, ‘b’ ]
Object.values(obj);
// [1, 2]
Object.entries(obj);
// [ [‘a’, 1], [‘b’, 2] ]
[/code]
String.prototype.padStart and String.prototype.padEnd are two simple string methods to pad out the left or right side of a string with spaces or other characters. Not having these built-in has had some rather dire consequences recently as a module implementing a similar capability was removed from NPM. Having these simple string padding APIs available in the standard library and avoiding the additional dependency (or bugs) will be very helpful to a lot of developers.
Chakra is also previewing SIMD, with the entire API surface area in the stage 3 SIMD proposal completed, as well as a fully-optimized SIMD implementation integrated with asm.js. We are eager to hear feedback from you on how useful these APIs are for your programs.
You can try ES2015 modules and other new ES2015, ES2016, and beyond features in Microsoft Edge, starting with the latest Windows Insider Preview and share your thoughts and feedback with us on Twitter at @MSEdgeDev and @ChakraCore or file an issue on the ChakraCore GitHub. We look forward to hearing your input!
― Taylor Woll, Software Engineer
― Limin Zhu, Program Manager
― Brian Terlson, Program Manager